(no title)
ARLK
0.1.0
Action Recognition Library for Kinect
|
#include <math.h>
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <float.h>
#include <string.h>
#include <stdarg.h>
#include <limits.h>
#include <locale.h>
#include "svm.h"
Classes | |
class | Cache |
class | QMatrix |
class | Kernel |
class | Solver |
struct | Solver::SolutionInfo |
class | Solver_NU |
class | SVC_Q |
class | ONE_CLASS_Q |
class | SVR_Q |
struct | decision_function |
Macros | |
#define | INF HUGE_VAL |
#define | TAU 1e-12 |
#define | Malloc(type, n) (type *)malloc((n)*sizeof(type)) |
Typedefs | |
typedef float | Qfloat |
typedef signed char | schar |
Functions | |
svm_model * | svm_train (const svm_problem *prob, const svm_parameter *param) |
void | svm_cross_validation (const svm_problem *prob, const svm_parameter *param, int nr_fold, double *target) |
int | svm_get_svm_type (const svm_model *model) |
int | svm_get_nr_class (const svm_model *model) |
void | svm_get_labels (const svm_model *model, int *label) |
void | svm_get_sv_indices (const svm_model *model, int *indices) |
int | svm_get_nr_sv (const svm_model *model) |
double | svm_get_svr_probability (const svm_model *model) |
double | svm_predict_values (const svm_model *model, const svm_node *x, double *dec_values) |
double | svm_predict (const svm_model *model, const svm_node *x) |
double | svm_predict_probability (const svm_model *model, const svm_node *x, double *prob_estimates) |
int | svm_save_model (const char *model_file_name, const svm_model *model) |
svm_model * | svm_load_model (const char *model_file_name) |
void | svm_free_model_content (svm_model *model_ptr) |
void | svm_free_and_destroy_model (svm_model **model_ptr_ptr) |
void | svm_destroy_param (svm_parameter *param) |
const char * | svm_check_parameter (const svm_problem *prob, const svm_parameter *param) |
int | svm_check_probability_model (const svm_model *model) |
void | svm_set_print_string_function (void(*print_func)(const char *)) |
Variables | |
int | libsvm_version = LIBSVM_VERSION |
Macro Definition Documentation
#define INF HUGE_VAL |
#define Malloc | ( | type, | |
n | |||
) | (type *)malloc((n)*sizeof(type)) |
#define TAU 1e-12 |
Typedef Documentation
typedef float Qfloat |
typedef signed char schar |
Function Documentation
const char* svm_check_parameter | ( | const svm_problem * | prob, |
const svm_parameter * | param | ||
) |
int svm_check_probability_model | ( | const svm_model * | model | ) |
void svm_cross_validation | ( | const svm_problem * | prob, |
const svm_parameter * | param, | ||
int | nr_fold, | ||
double * | target | ||
) |
void svm_destroy_param | ( | svm_parameter * | param | ) |
void svm_free_and_destroy_model | ( | svm_model ** | model_ptr_ptr | ) |
void svm_free_model_content | ( | svm_model * | model_ptr | ) |
void svm_get_labels | ( | const svm_model * | model, |
int * | label | ||
) |
int svm_get_nr_class | ( | const svm_model * | model | ) |
int svm_get_nr_sv | ( | const svm_model * | model | ) |
void svm_get_sv_indices | ( | const svm_model * | model, |
int * | indices | ||
) |
int svm_get_svm_type | ( | const svm_model * | model | ) |
double svm_get_svr_probability | ( | const svm_model * | model | ) |
|
read |
double svm_predict_probability | ( | const svm_model * | model, |
const svm_node * | x, | ||
double * | prob_estimates | ||
) |
int svm_save_model | ( | const char * | model_file_name, |
const svm_model * | model | ||
) |
void svm_set_print_string_function | ( | void(*)(const char *) | print_func | ) |
svm_model* svm_train | ( | const svm_problem * | prob, |
const svm_parameter * | param | ||
) |
Variable Documentation
int libsvm_version = LIBSVM_VERSION |
Generated on Fri May 31 2013 10:57:38 for ARLK by
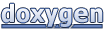